Java Collections
As most applications deal with a list of data types, the Java Collections API is one of the most essential tools for programming in Java.
The Collection Interface
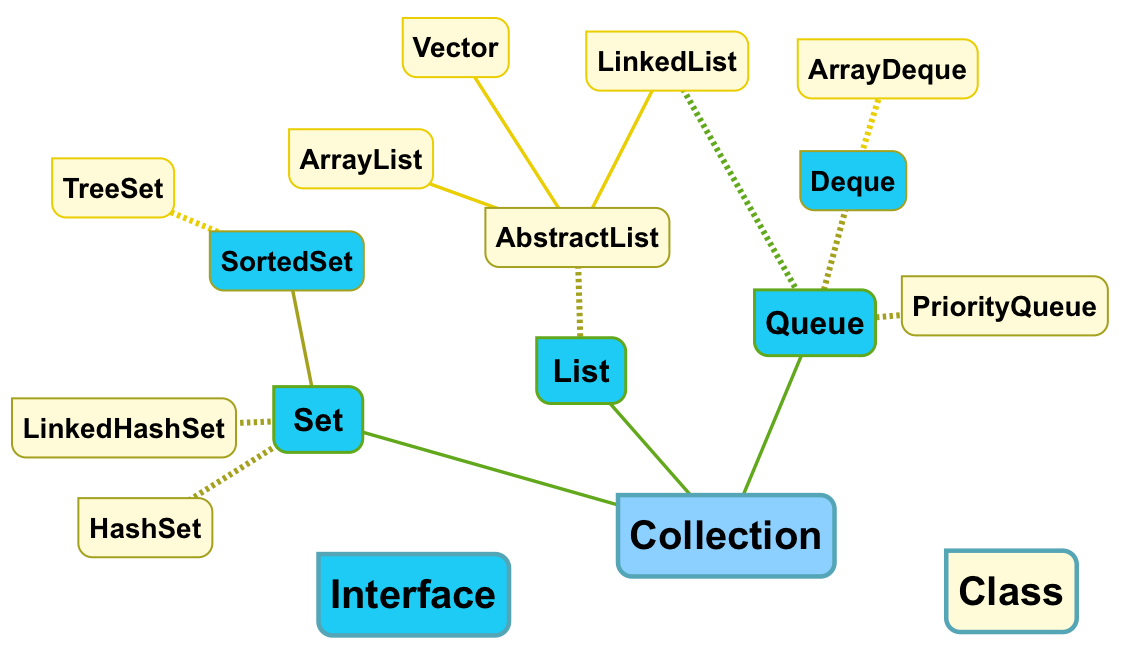
A Collection is a grouping of objects.
A Set is a type of Collection with no duplicates.
A List is a Collection of ordered elements that may contain duplicates.
For collections to work properly, you must properly define the
hashCode()
andequals()
methods described in Inherited methods from Object().For general usage, the ArrayList provides the best all-round implementation for the various methods in the Collection class.
The Vector and Stack are legacy collections and should not be used.
The Queue Interfaces
A queue is an ordered collection of elements, with methods for extracting elements in order, from the head of the queue.
Queue can be FIFO and LIFO. The Deque is a LIFO interface.
Unlike a Set, a Queue typically allows duplicate elements.
The Deque (Double Ended Queue) interface is a linear collection that supports element insertion and removal at both ends.
The ArrayDeque class provides the features of Deque and resizeable arrays.
Unlike List, the Queue interface does not define methods for manipulating its elements at arbitrary positions. Only the element at the head of the queue is available for examination.
The
peak()
andpoll()
methods return null to indicate a queue is empty. Hence, most Queue implementations do not allow null elements.The LinkedList class implements the Queue interface to provide unbounded FIFO ordering.
The PriorityQueue orders its elements according to a Comparator or orders Comparable elements according to the defined
ComapreTo()
methods.
The BlockingQueue Interface
The blocking queue defines blocking
put()
andtake()
methods.The
put()
method adds an element to the queue, waiting if necessary until there is space in the queue for the element.
The Map Interface
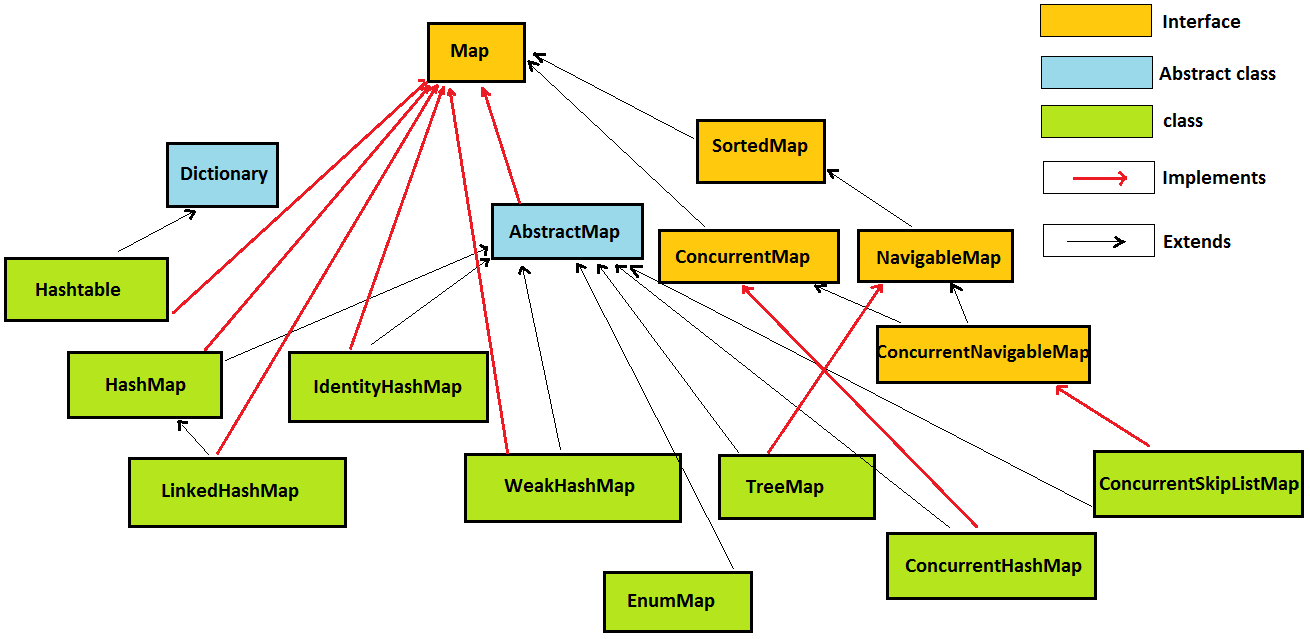
A Map is a set of mappings or associations between objects. The more important methods of Map are:
put
: which defines a key/value pair in the mapget
: which queries the value associated with a specific keyremove
: which removes the specified key and its associated value from the map.
The important features of a Map can be summarised as:
A Map is not a Collection.
The keys of a Map can be viewed as a Set.
The values of a Map can be viewed as a Collection.
The mappings can be viewed as a Set of
Map.Entry
objects. This is a nested interface defined within Map to represent a single key/value pair.
Collection Utility Methods
The Java Collections class provides a number of static utility methods designed to be used with collection. Many of them are wrapper methods. They return a special purpose collection wrapper around a collection that you specify.
The first set of utility classes provide threadsafe wrappers around collections.
List<String> list = Collections.synchronizedList(new ArrayList<>());
Set<Integer> set = Collections.synchronizedSet(new HashSet<>());
Map<String,Integer> map = Collections.synchronizedMap(new HashMap<>());
The second set of wrapper methods provide unmodifiable collection objects with a read-only view of the collection. This provides many of the benefits of Useful immutable types.
List<Integer> primes = new ArrayList<>();
List<Integer> readonly = Collection.unmodifiableList(primes);
Some other more interesting and possibly useful Collection methods are given below.
Collections.copy(list1, list2); // Copy list2 into list1, overwritting list1
Collections.fill(list, a); // Fill list with Object o
Collections.max(c); // Get the largest element
Collections.min(c); // Get the smallest element
Collections.reverse(list); // Reverse the list
Collections.shuffle(list); // Shuffle the list
// nCopies() returns an immutable List containing copies of a specified object
List<Integer> tenzeros = Collections.nCopies(10,0);
Take advantage of Java Streams
By using a collection’s stream()
method, you can easily take advantage of Java Functional Programming.