Java Data Types
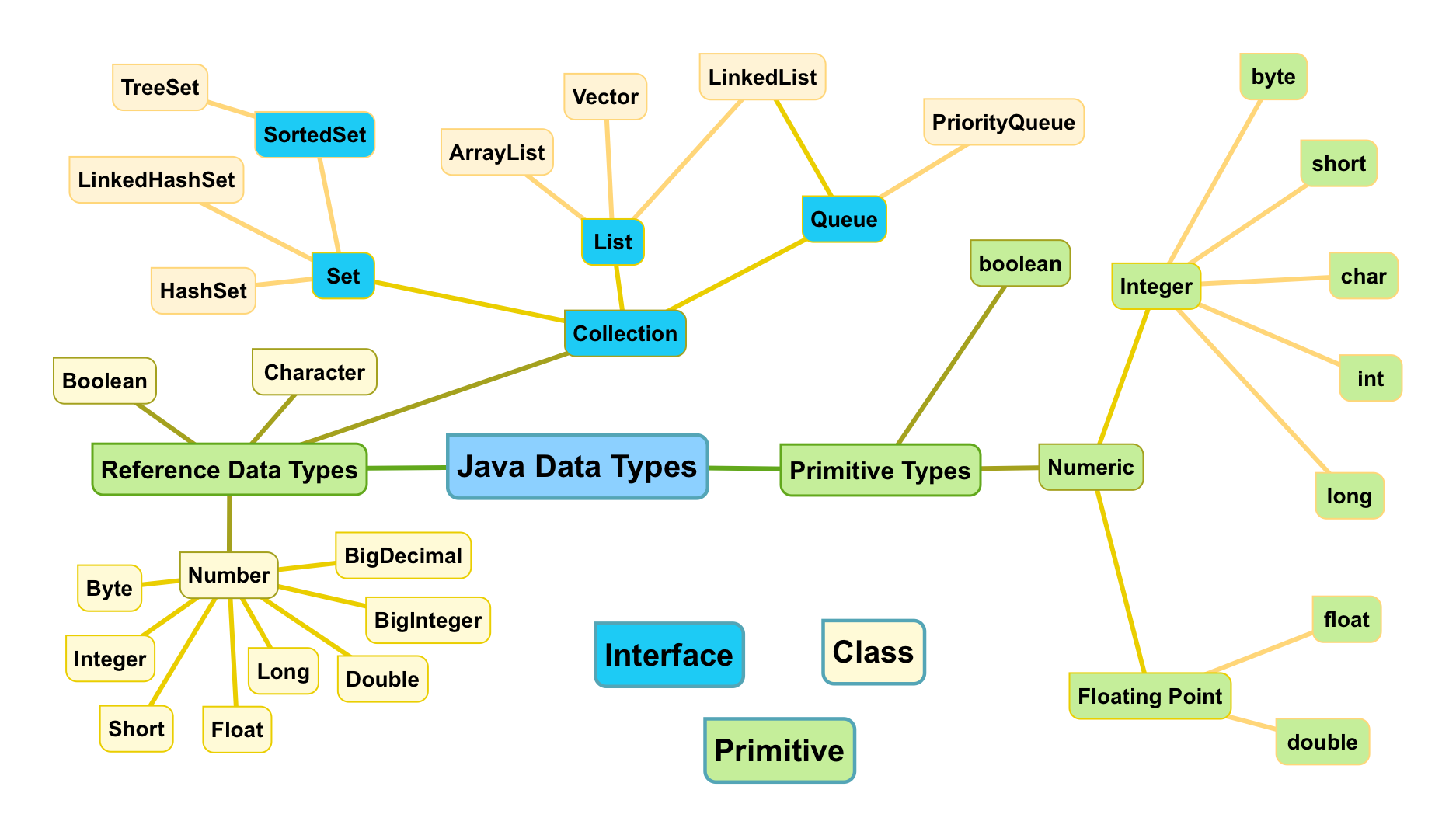
Primitive Data Types
The primitive data types available are byte, short, int, long, float, double, boolean and char.
Boxing and Unboxing
Each primitive data type has a corresponding Reference Data Type, also known as its boxed type.
Java provides a wrapper class for each of these primitive types to be boxed or unboxed into their corresponding reference type.
These boxed reference types are immutable final classes, usually used to store single primitive values in collections such as
java.util.List
.Boxing and unboxing are usually automatically performed by Java where necessary.
byte –> Byte
short –> Short
int –> Integer
long –> Long
float –> Float
double –> Double
boolean –> Boolean
char –> Character
Reference Data Types
Classes, interfaces, arrays, enumerated types and annotations are Java’s reference types.
New primitive types cannot be defined, whereas new reference types can be user defined.
Reference types can hold zero or more primitive type values or objects.
BigDecimal
is an exact way of representing decimal numbers compared toDouble
, which has a certain precision. Hence, when dealing with money, its better to useBigDecimal
.
Comparing Objects
With reference types,
==
compares references and not actual objects, i.e. whether they refer to the same object.It does not test whether the two objects have the same content.
All objects inherit an
equals()
method from the Objects class. Its default implementation compares object references rather than object content.
Automatic Boxing and Unboxing examples
Integer i = 0 // the literal zero boxed to an Integer object
int j = 1; // i is automatically unboxed
i++; // i is unboxed, incremented and boxed up again
Integer k = i+2; // i is unboxed and the sum boxed up again
i = null;
j = i; // Because i is null, this assignment throws a NullPointerException
Pass By Value
Java is a pass-by-value language as compared to pass-by-reference in C and C++.
In Java, when a reference type is involved, the value that is passed is a copy of the reference, which is not the same as pass-by-reference.
public void manipulate(Circle circle) {
circle = new Circle(3);
}
Circle c = new Circle(2);
manipulate(c);
System.out.println("The radius of c is " + c.getRadius());
If Java was a pass-by-reference language, the reference
c
would point toCircle(3)
However, it still points to
Circle(2)
because Java creates a copy ofc
orCircle(2)
and passes this new reference to themanipulate
method.Hence, what is printed out in the above code for the radius is 2 instead of 3.
Inherited methods from Object()
All classes directly or indirectly extend the
java.lang.Object
class.This class defines a number of useful methods that were designed to be overridden by the your user-defined class:
toString(): provides a textual representation of an object. Especially useful for debugging and logging outputs.
equals(): compares two references to see if they refer to the same object. Should be overridden for the right implementation of comparing two different objects of the same class. If it is overridden, the
hashCode()
method must also be overridden to guarantee that equal objects have equal has codes. Failing to do so can cause subtle bugs in your application.hashCode(): returns an integer representation of the object. It works with the
equals()
method. Hence, it is important that two objects that are equal, have the same hashCode representation. This is because the are used by hash table data structures.
Another equally important method that is not extended by the
java.lang.Object
class is thecompareTo()
method. It is recommended to implement this method, especially for sorting. - Comparable::compareTo(): This method, defined by thejava.lang.Comparable
interface allows instances of a class to be compared to each other in a similar way to <, <=, > and >= operators. It allows for the class to be sorted.
Interfaces
interface Centered {
void setCentre(double x, double y);
double getCentreX();
double getCentreY();
}
The above example shows how an interface can be defined where all methods are implicitly abstract.
It does not define any instance fields, except for constants that are declared both static and final.
Generic
interface Box<T> {
void box(T t);
T unbox();
}
The above example show a generic data type T which can be replaced with any Reference Data Type, such as String and Integer.
Constants
import static Constants.PLANCK_CONSTANT;
import static Constants.PI;
public class Calculations {
public double getReducedPlanckConstant() {
return PLANCK_CONSTANT / (2 * PI);
}
}
To access constants without having to fully qualify them (i.e. without having to prefix them with the class name), use a static import (since Java 5).
Excessive use of static imports can actually make your code less readable. Just use
static final int MAX_UNITS = 25;
or something similar in your class instead.
Instance Fields
public class Point {
private int x, y;
public Point(int pX, pY) {
x = pX;
y = pY;
}
public void setPoint(int pX, pY) {
x = pX;
y = pY;
}
public Point getX() {
return x;
}
public Point getY() {
return y;
}
}
An instance field is a variable that’s bound to the object itself and accessible only through getter and / or setter methods, as shown above.