Cross Origin Resource Sharing (CORS)
Introduction
CORS is something that many people do not have a good grasp on, especially me. It is only recently that I delved into finding out more about CORS before I started to understand what it was and how it is used.
These are my notes on CORS.
Concepts
If you wanted to create a web application to call an external API, e.g., a HTML page to display photos, where you add JavaScript code to load photos from a Flickr page, it would not work.
This is because by default, the browser’s same-origin policy, limits client code from making HTTP requests to a different domain.
Cross-Origin Resource Sharing OR CORS, was built to solve this issue. It is a way of making HTTP requests from one place to another. This is difficult to do in JavaScript because, for years, the browser’s same-origin policy has explicitly prevented these types of requests.
This may make CORS seem like a contradiction, however, its only when you look into the details that you understand why it is not so. CORS allows server to dictate who can make requests to them and what kind of requests are allowed. A server has the choice to open up its APIs to all clients, only to a small number of clients, or prevent access to all clients. But, how is this done? The secret lies in the request and response headers between the client web application and the server.
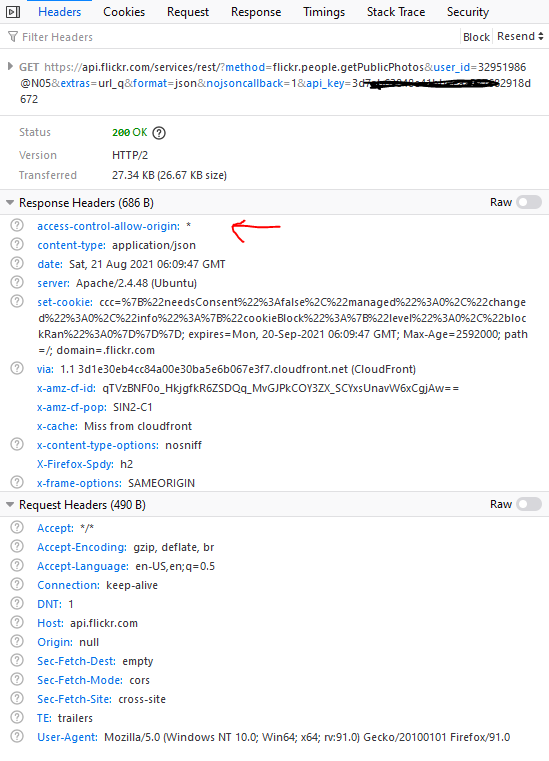
A request to the server with the server’s response
In the diagram above, we can see the request being sent to the server api.flickr.com and the server responding with access-control-allow-origin: *
which allows any client to connect to this API.
CORS Server Options
CORS gives the server flexibility to configure cross-origin access in a variety of ways. These include:
Which domains are allowed to make requests
Which HTTP methods are allowed (e.g., GET/PUT/POST/DELETE)
Which headers are allowed on the HTTP request
Whether or not requests may include cookie data
Which response headers the client can read
With these options provided above, the API server is in control of how the CORS requests work.
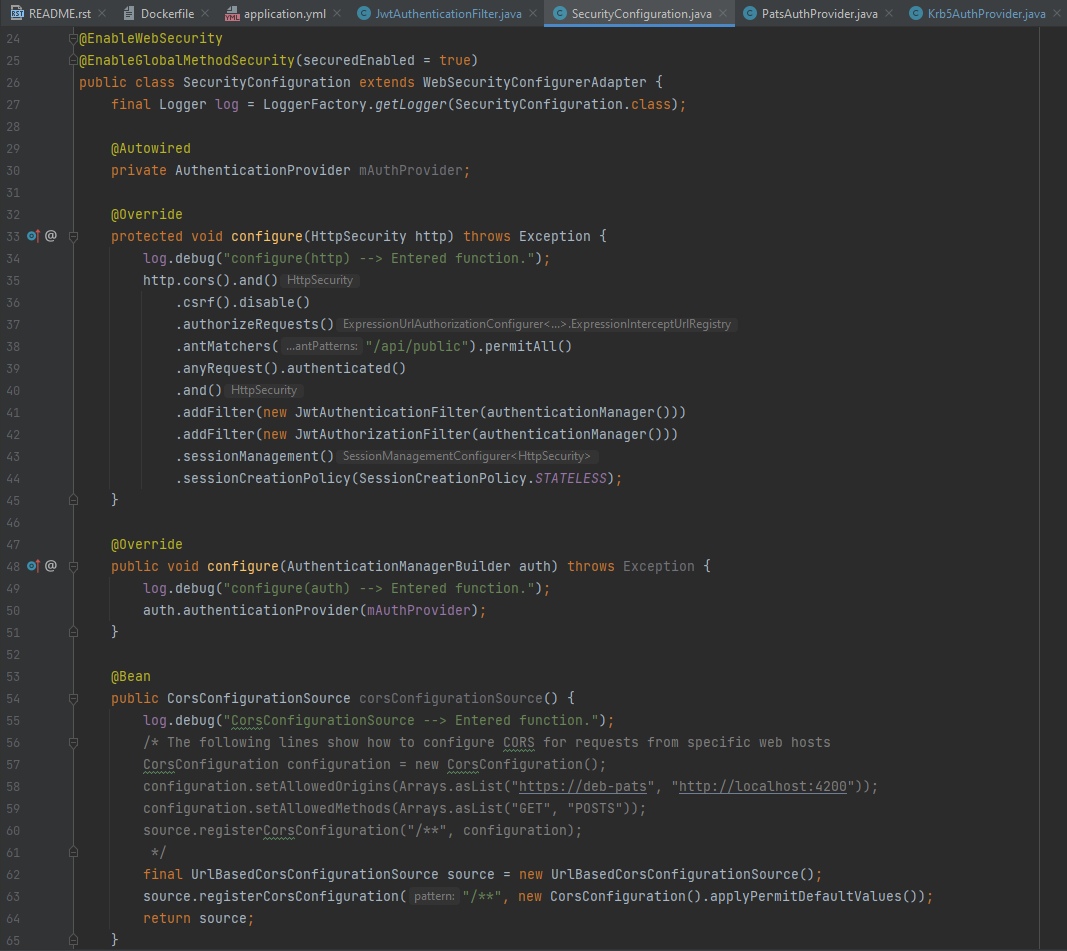
Spring Boot configuration of CORS
In the diagram above which shows a Java Spring Boot application, the Spring Boot web framework allows configuration of CORS in the corsConfigurationSource() method from lines 54 onwards. In this case, we have allowed requests to come in from any web server in line 63.