Spring Boot Persistence
Transaction Management
A transaction refers to a series of operation which are treated as a single unit. These operations must all succeed, or they should all not go through. They have the following ACID characteristics:
A tomicity: Either all operations in a transaction succeed or none of them succeed.
C onsistency: The resulting state after the transaction is that all data in the system is valid.
I solation: Changes made within a transaction are only visible to the transaction making this change.
D urability: The changes made endure beyond the completion of the transaction.
Relationship Concepts
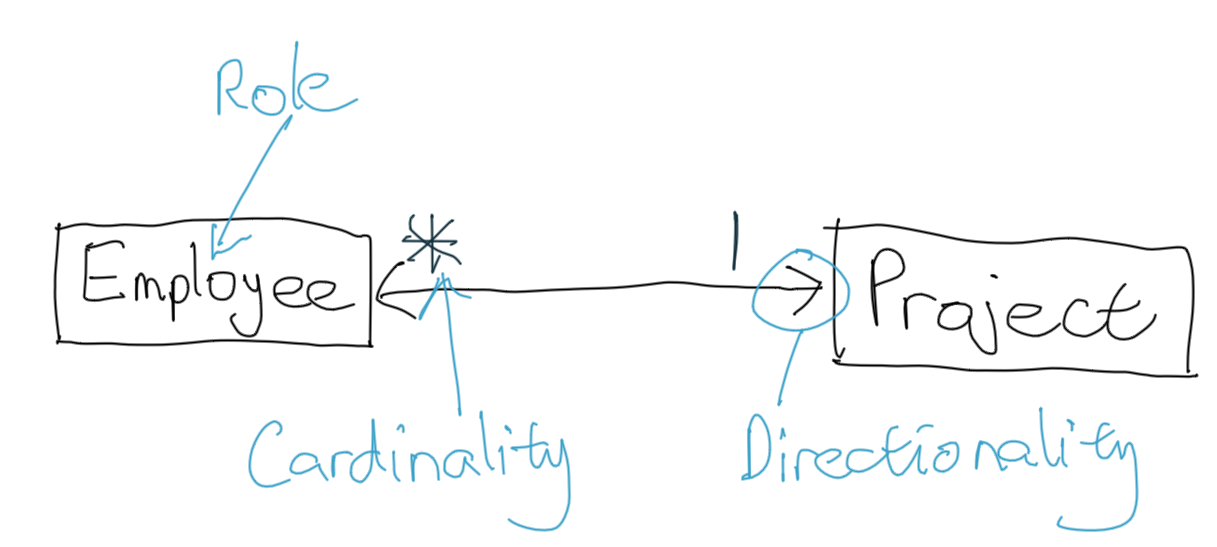
Role: The role of the given entity and what it stores
Directionality: In the above example, the employee entity has a link to Project and vice versa, i.e. the employee entity has a field to store the project the employee works for. The Project entity stores the list of employees in the project. To Note: It is generally recommended to use a bi-directional association to make it easier to navigate the object graph in either direction.
Cardinality: If this is a OneToOne, OneToMany or ManyToMany relationship. Ordinality defines if this entity exists or not, i.e., a 0 or 1 ordinality.
Object Mapping
mappedBy
In a @OneToMany, @ManyToOne or @ManyToMany object mapping, the lack of the mappedBy keyword on the join column denotes that this table and column is the owner of the relationship.
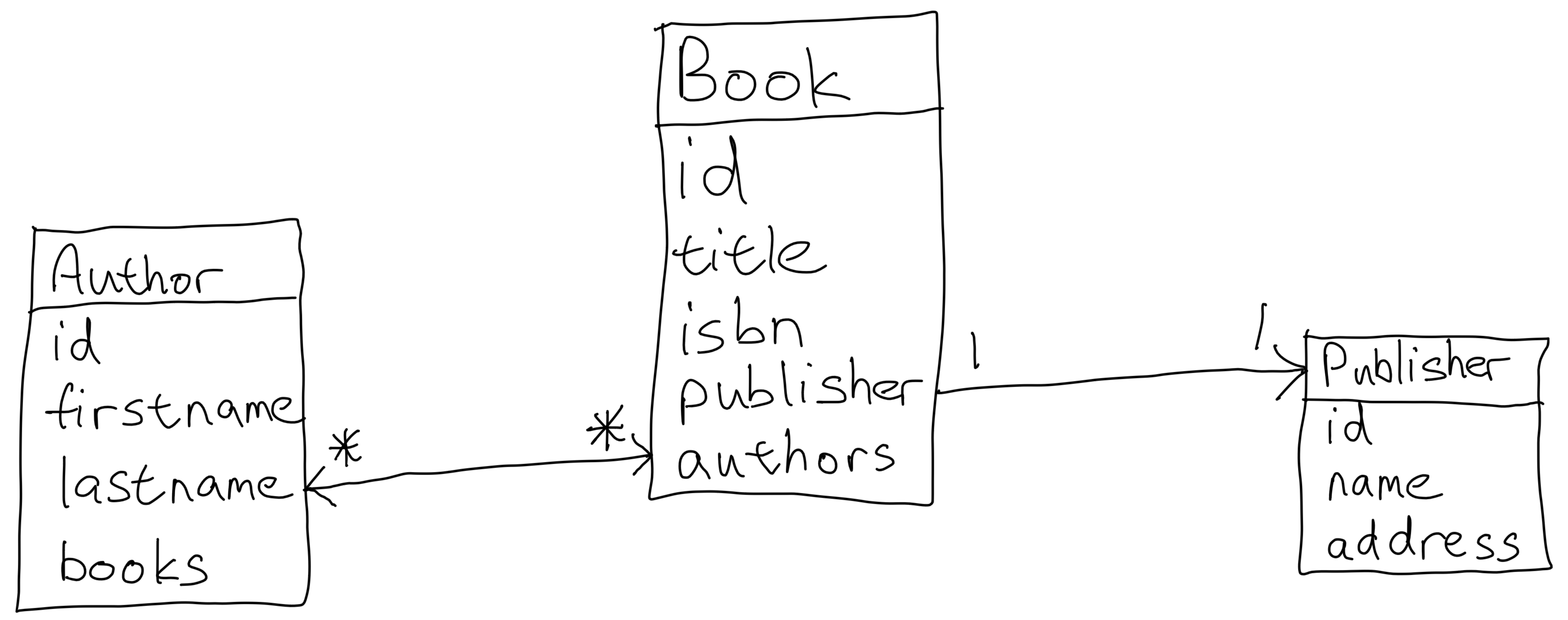
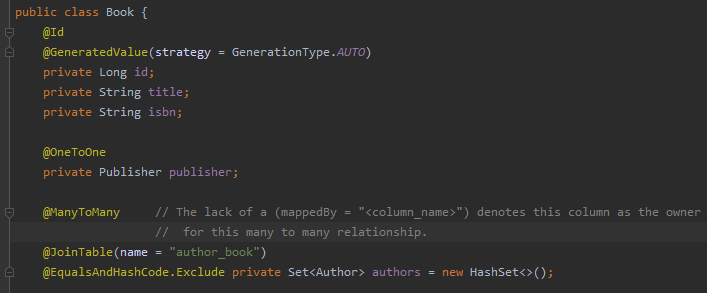
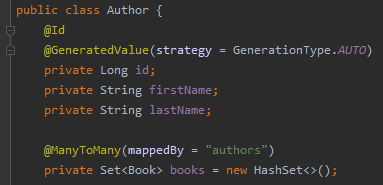
Here, the mappedBy parameter is required and used in the relationship to let the compiler know that the Author object does not own this relationship and that the authors field (from the Book object) is the owner field.
This books field in the Author object is purely a reference back to the Books.authors field. It will not be created in the database table Author as it is not the owner.
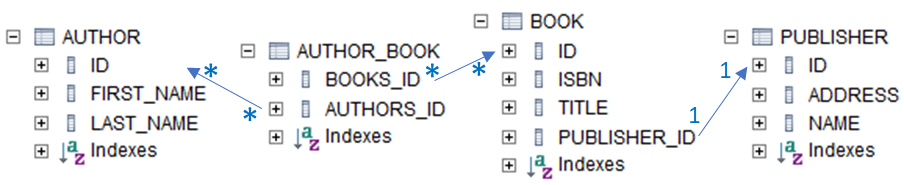
The diagram above shows the resultant table structure produced by the Spring JPA library with the provided object model on a H2 Database.
Fetch Type
Lazy: Data is not queried until referenced
Eager: Data is queried up front
Hibernate 5 JPA 2.1 fetch type defaults are:
OneToMany – Lazy
Many to One - Eager
ManyToMany – Eager
OneToOne – Eager
Cascade Types
JPA Cascade Types control how state changes are cascaded from the parent object to the child objects.
PERSIST – save operations will cascade to related entities
MERGE – related entities are merged when the owning entity is merged
REFRESH – related entities are refreshed when the owning entity is refreshed
REMOVE – removes all related entities when the owning entity is deleted
DETACH – detaches all related entities if a manual detach occurs
ALL – applies all the above cascade options
By default, no operations are cascaded
Inheritance
MappedSuperclass – entities inherit from a super class. A database table IS NOT created for the super class.
Single Table – (Hibernate Default) where one table is used for all sub classes
Joined Table – base class and sub classes have their own tables. Fetching sub class entities require a join to the parent table
Table Per Class – Each sub class has its own table.
JPA Auto Generated Values
Marking a field as @GeneratedValue denotes that a value will be automatically generated for that field.
AUTO – This is the default strategy which uses the global number generator to generate a primary key for every new entity object. Generated values are unique for the database.
IDENTITY – This is also automatically generated, but a separate identity generator is managed per type hierarchy. Generated values are unique to that entity type.
SEQUENCE – Consists of two parts:
Name Sequence – Define a sequence and accepts a name, an initial value and an allocation size. It is global to the application and can be used by one or more fields in one or more classes.
Using named sequence – Use the defined named sequence method in the entity’s ID field.
TABLE – Equivalent to SEQUENCE but instead uses a table to support this strategy.
Spring Data Repositories
JpaRepository –> PagingAndSortingRepository –> CrudRepository
CrudRepository is the most basic Spring data repository implementation which provides CRUD functions.
PagingAndSortRepository provides methods to do pagination and sorting of records.
JpaRepository provides some JPA related methods such as flushing the persistence context and deleting records in a batch.
JpaRepository have all the functions of CrudRepository and PagingAndSortingRepository. So if you just need basic functionality, use CrudRepository.